Setting up a React Project From Scratch
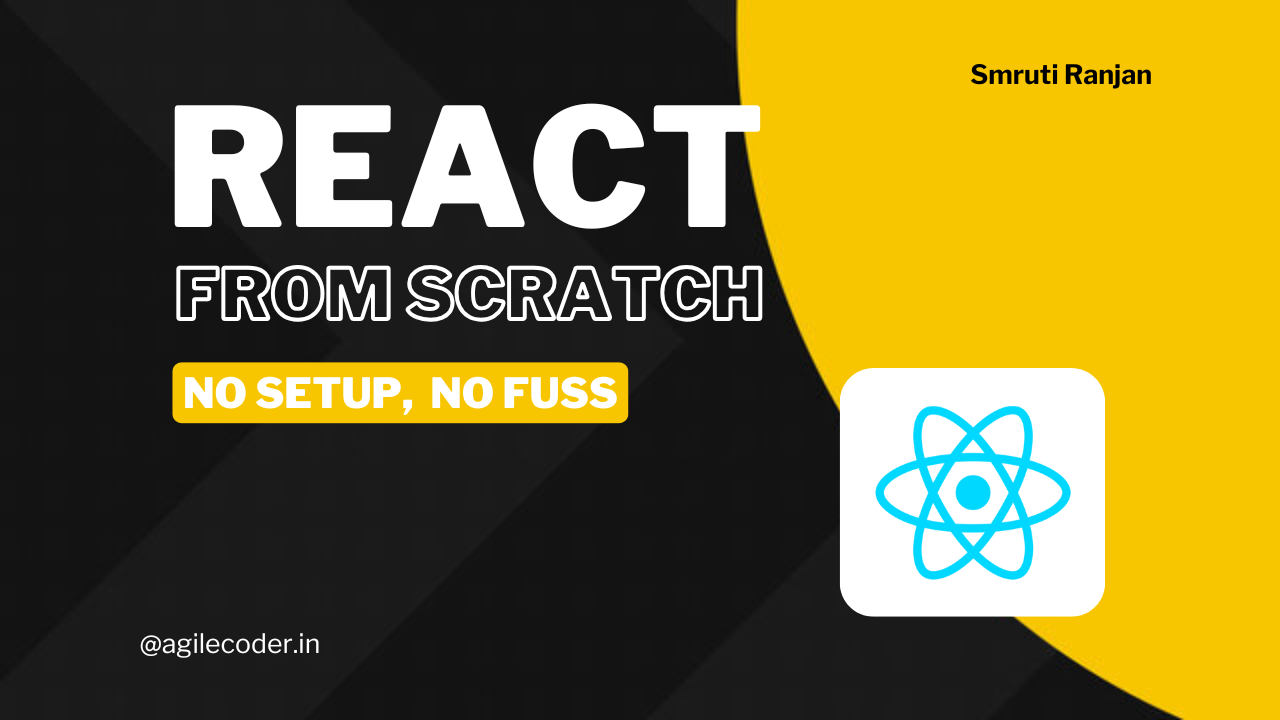
If you know HTML and JavaScript, you’ve likely created a simple webpage like this:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>A Boring HTML Page</title>
</head>
<body>
<h2>Hello World</h2>
</body>
</html>
But what if we want to add React to this page? Since we can include additional libraries using the <script>
tag, we can integrate React by adding two essential libraries:
react
react-dom
Adding React to a Simple HTML Page
Here’s how you can add React using a CDN:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>React Bare Setup</title>
<script src="https://unpkg.com/react@18/umd/react.development.js" crossorigin></script>
<script src="https://unpkg.com/react-dom@18/umd/react-dom.development.js" crossorigin></script>
</head>
<body>
<div id="app"></div>
<script src="index.js"></script>
</body>
</html>
Now, let’s create an index.js
file to write our React code:
const rootElement = document.getElementById('app');
const root = ReactDOM.createRoot(rootElement);
root.render(
<h2>Hello World</h2>
);
The Problem with JSX
The code above uses JSX (JavaScript XML), which is not natively supported by browsers. JSX needs to be transpiled into plain JavaScript before the browser can execute it.
To understand this better, here’s how JSX works under the hood:
const rootElement = document.getElementById('app');
const root = ReactDOM.createRoot(rootElement);
root.render(
React.createElement("h2", null, "Hello World")
);
This works because React.createElement()
is a function that generates the necessary JavaScript representation of the component.
Transpiling JSX with Babel
To use JSX directly, we need to add Babel to our HTML file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>React Bare Setup</title>
<script src="https://unpkg.com/react@18/umd/react.development.js" crossorigin></script>
<script src="https://unpkg.com/react-dom@18/umd/react-dom.development.js" crossorigin></script>
<script src="https://unpkg.com/@babel/standalone/babel.min.js"></script>
</head>
<body>
<div id="root"></div>
<script type="text/babel" src="index.js"></script>
</body>
</html>
Now, we can write JSX in index.js
and it will work fine:
const rootElement = document.getElementById('root');
const root = ReactDOM.createRoot(rootElement);
root.render(
<h2>Hello World</h2>
);
Creating a React Component
Now, let’s create a simple React component:
const rootElement = document.getElementById('root');
const root = ReactDOM.createRoot(rootElement);
const HelloComponent = () => {
return (
<h2>Hi There</h2>
)
}
console.log(HelloComponent);
root.render(
<HelloComponent />
);
If you check the console, you’ll notice something interesting. The HelloComponent
is just a JavaScript function! This proves that React components are nothing but JavaScript functions that return JSX.
Conclusion
With this setup, you’ve successfully created a React project from scratch without any build tools like Webpack or Vite. This is useful for quick prototyping or learning React without setting up a complex development environment.
For production, though, using a framework like Create React App or Vite is recommended for better performance and scalability.
Happy coding! 🚀