SEO for Next.js: How to Optimize Your Application for Search Engines
In this article, learn how to optimize SEO in Next.js by managing metadata and Open Graph properties for better social media previews. We also cover the importance of server-side rendering to boost search engine ranking and engagement, using simple yet effective techniques.
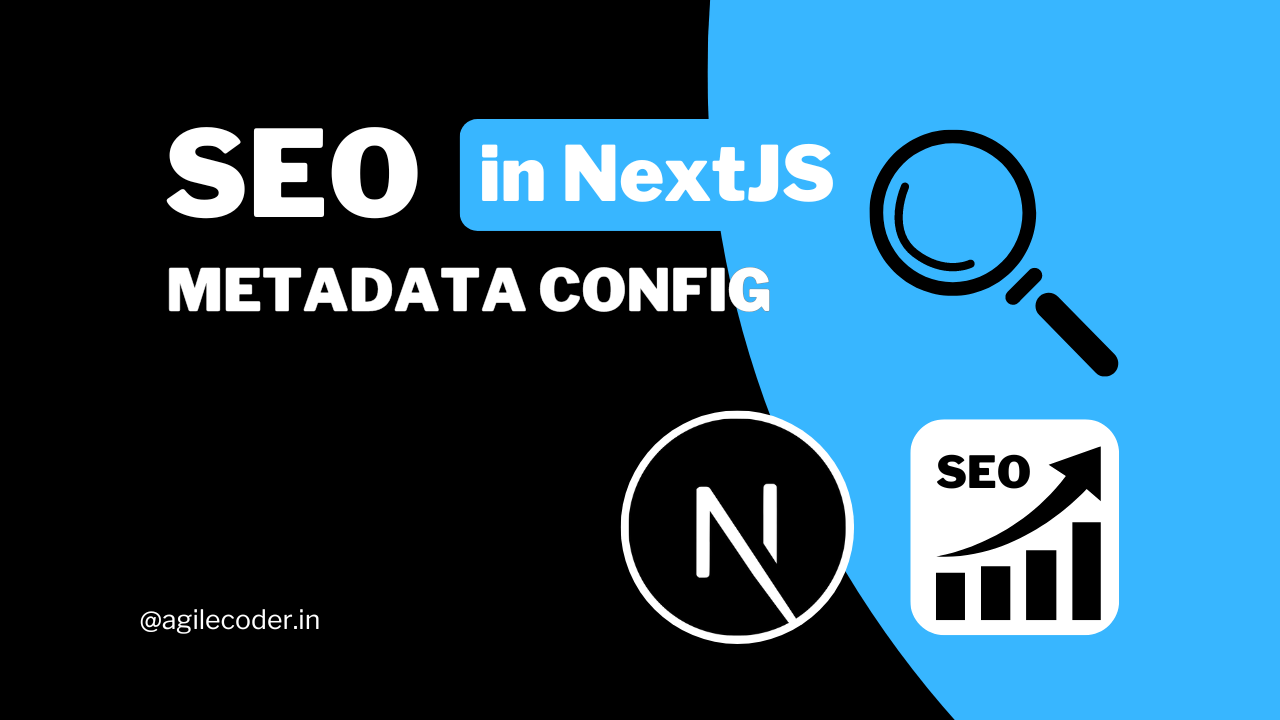
In today's competitive online environment, search engine optimization (SEO) is crucial for the success of any website. Modern frameworks like Next.js offer developers robust tools to ensure their websites are SEO-friendly. By managing metadata effectively, generating dynamic sitemaps, and controlling site access through a robots.txt
file, you can significantly improve how search engines index and rank your site.
There are three key areas of SEO for Next.js applications:
- Metadata Management (including Open Graph for social media)
- Dynamic Sitemap Generation
- Managing
robots.txt
In this article, we will discuss Metadata Management. I will be posting articles for the other two as well, stay tuned ✌️
Metadata Management for SEO
Metadata refers to the information embedded in your web pages that provides details about the content to search engines and social media platforms. In websites, we do this using <meta>
tags.
This information is vital for SEO because it helps search engines understand your pages' structure, relevance, and context. Additionally, platforms like Facebook and Twitter use metadata to create rich previews when your links are shared, making the content more engaging and clickable.
To optimize SEO in Next.js using the metadata
object, you can use the new app
directory structure (introduced in Next.js 13) provides a more structured and declarative way to manage metadata for each page or layout.
To add metadata to a page in Next.js, you can define it like this:
export const metadata = {
// metadata information
}
const BlogPage = () => {
return (
// component
)
}
In Next.js, it's important to ensure that your pages are rendered on the server side to take full advantage of SEO optimizations. Rendering on the server allows search engines to easily access your metadata, which improves how your site is indexed.
Next.js offers a directive called "use client"
. This directive specifies that the component should be rendered in the browser, allowing you to manage dynamic interactions or states.
Here’s an example of a client-side component in Next.js:
"use client"
const BlogPage = () => {
return (
// component
)
}
metadata
object. Metadata is designed to be part of server-side rendering to ensure it’s available for search engines and social platforms during the initial page load. Client-side components don't have this capability because they are rendered on the browser, after the page has already loaded.If you have client-side code on your page component i.e. you have used things like useState, useClient, and other hooks. These are client-side codes, for this, you can do something like this
📂 app
┣ 📂 blog
┃ ┣ 📂 [slug]
┃ ┃ ┣ 📜 page.jsx # Server-side component with metadata
┃ ┃ ┣ 📜 BlogPage.jsx # Client-side component
Then you can easily structure your static pages, with dynamic client-side code.
// Server-side component where metadata is handled
import BlogPage from './BlogPage'; // client-side component
export const metadata = {
// Metadata Informations
};
const BlogPage = () => {
return (
<div>
{/* Render client-side component */}
<BlogPage />
</div>
);
};
export default BlogPage;
page.jsx
"use client"; // This makes the component a client-side component
const ClientBlogPage = () => {
// Client-side logic or interactive content
return (
<div>
<p>This part of the page is rendered on the client side.</p>
</div>
);
};
export default ClientBlogPage;
BlogPage.jsx
With this approach, you can easily manage metadata for each page and tailor it to enhance your SEO performance. But what kind of metadata should you add?
Important Metadata Properties for SEO
When optimizing for SEO, there are several important metadata properties you can include. Here’s an example that includes the most important ones
export const metadata = {
title: 'My Blog Post',
description: 'An in-depth blog post about web development',
openGraph: {
type: 'article',
title: 'My Blog Post Title',
description: 'An in-depth blog post about web development',
url: 'https://example.com/blog/my-post',
images: [
{
url: 'https://example.com/images/post-image.jpg',
width: 1280,
height: 720,
alt: 'A description of the image',
},
],
siteName: 'Example Site'
},
};
Next.js automatically includes some of the most common meta tags for you, such as:
<meta name="viewport" content="width=device-width, initial-scale=1" />
<meta charset="UTF-8" />
This means you don’t have to worry about adding them manually, saving you time and effort. With these essential tags handled for you, you can focus on optimizing other important aspects of your application!
Also, you can add properties for X ( formerly Twitter)
Open Graph (OG) Properties for Social Media Previews
Open Graph (OG) properties are essential for shaping how links from your website appear when shared on social media platforms like Facebook, LinkedIn, and even Slack. These rich previews include elements such as:
- Title: The title of the content.
- Description: A summary of what the content is about.
- Image: An eye-catching image that represents the content.
You can check your Open Graph properties using the tool at opengraph.xyz.
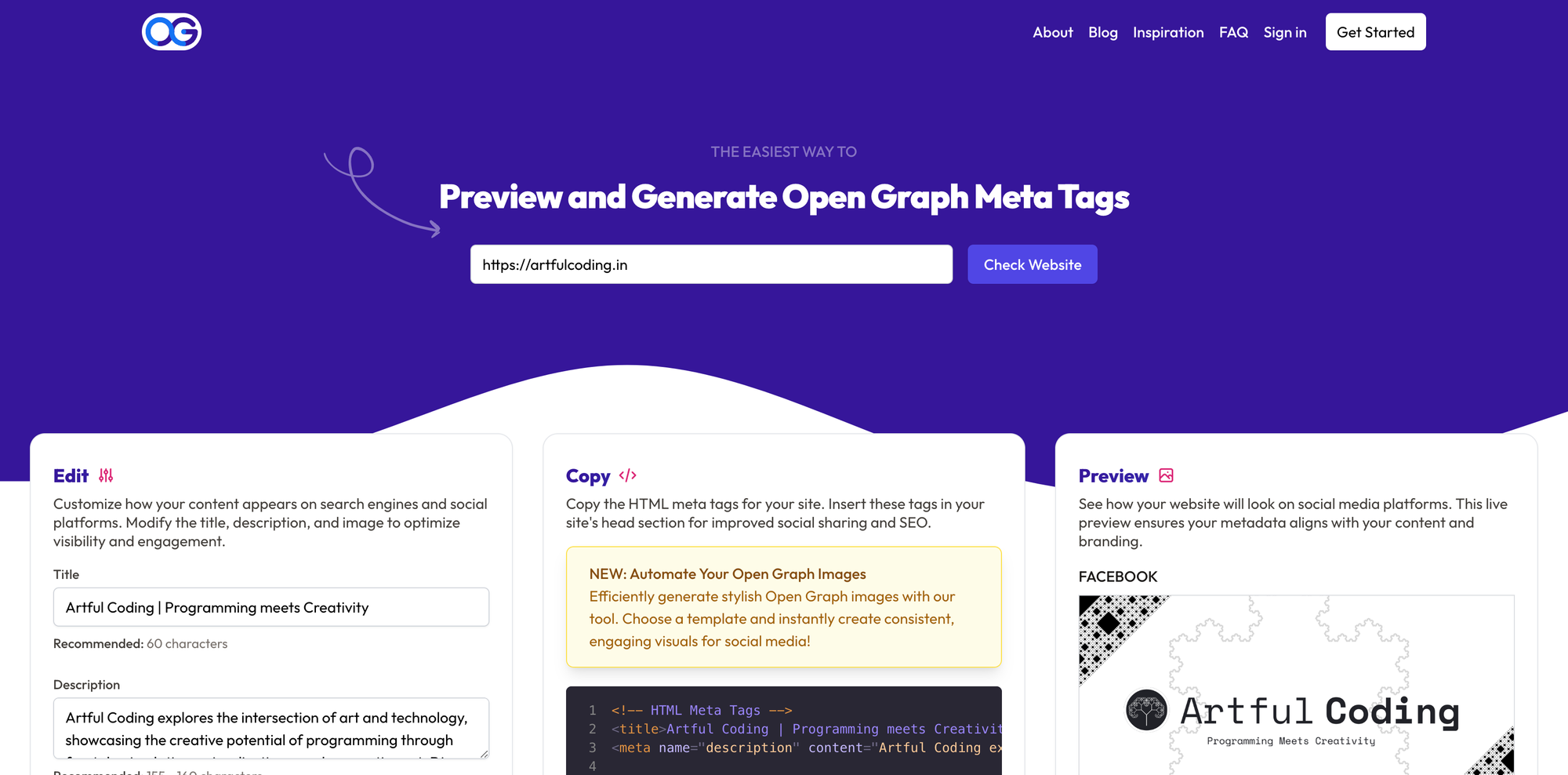
When Open Graph metadata is optimized correctly, it can increase user engagement exponentially by:
- Capturing attention: A well-designed preview with a catchy image and compelling description grabs the viewer’s attention quickly.
- Boosting click-through rates (CTR): A visually rich and informative preview encourages users to click on your link rather than scroll past it.
- Improving branding: Consistent use of branding elements like logos, images, and structured information in OG tags helps solidify your brand identity.
For example, instead of a dull link with a random snippet of text, an Open Graph preview can display:
- A bold headline that captures the attention
- A descriptive summary that clearly explains what the content is about
- A relevant image that makes the content visually appealing
This combination not only makes your link more shareable but also builds trust and curiosity, encouraging users to engage with your content.
Below are some of the previews of artfulcoding.in for different social media platforms.
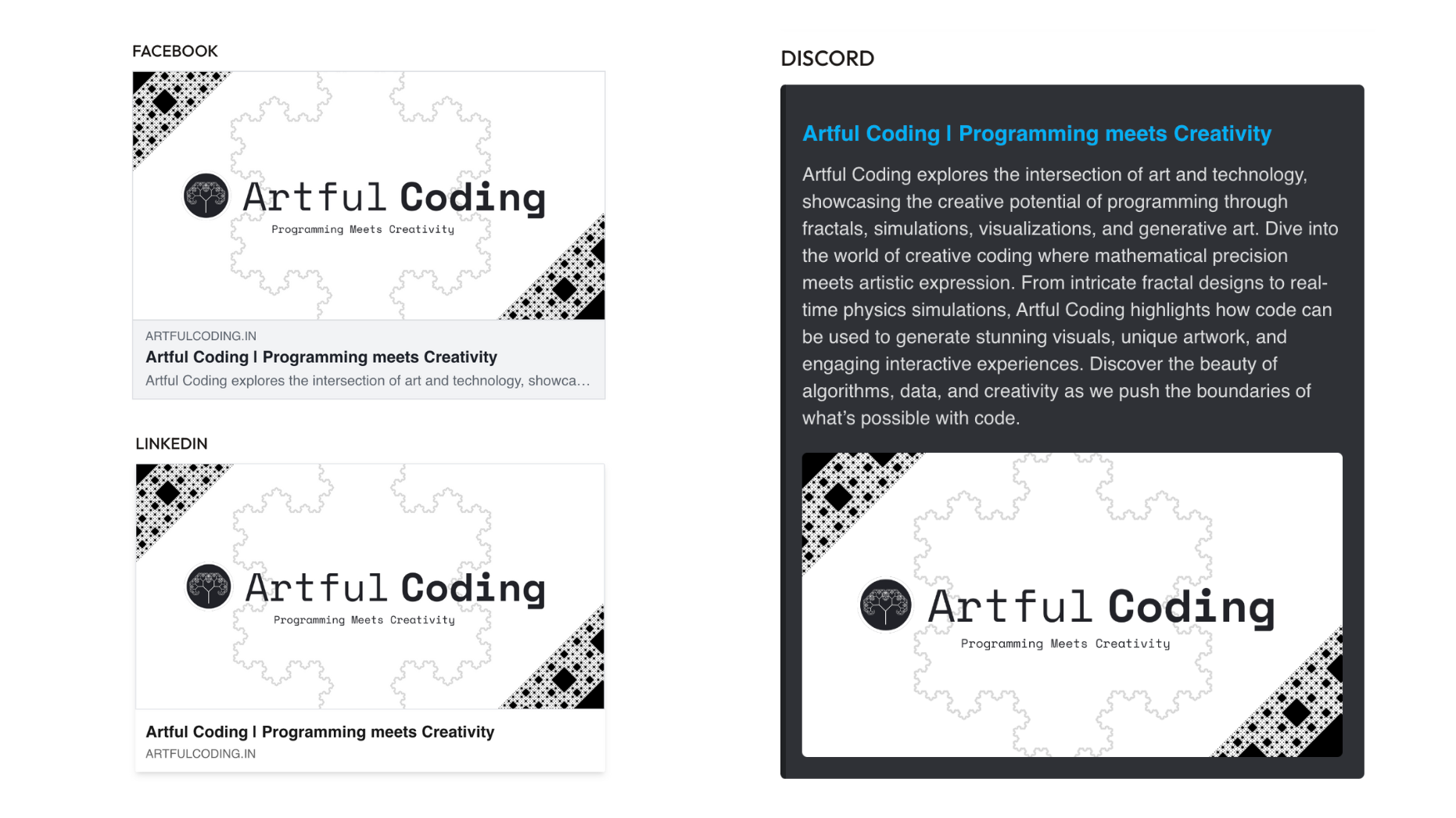
By ensuring that Open Graph metadata is set up for each page—especially for key content like blog posts, product pages, or events—you’re setting your site up for greater visibility and interaction on social media.
For businesses, bloggers, and content creators, these well-optimized previews can make the difference between a link being ignored or widely shared. When you provide social media platforms with clear and engaging metadata, you can expect:
- Higher engagement rates with your shared content.
- More clicks on your links.
- Improved brand recognition as your content gets consistently styled and shared.
Metadata management, especially using Open Graph properties, is a powerful tool for optimizing your website’s visibility and engagement on social media. In Next.js, adding and managing this metadata is straightforward, allowing you to take full control of how your content appears to search engines and social media users.
That's it for this article, feel free to ask me if you having trouble with your SEO. See ya 👋
FAQs
Can I use metadata in client-side components in Next.js?
No, metadata is designed to be part of the server-side rendering process in Next.js. It needs to be exported from a server-side component to be accessible by search engines and social media during the initial page load. Client-side components can't export metadata.
Does Next.js automatically add any common meta tags?
Yes, Next.js automatically adds common meta tags like <meta name="viewport" content="width=device-width, initial-scale=1">
and <meta charset="UTF-8">
, so you don’t need to add these manually.
How can I check if my Open Graph metadata is set up correctly?
You can use online tools like opengraph.xyz to validate your Open Graph tags and preview how your content will appear when shared on social media.