NodeJS: Explore the Core Features and Use Cases
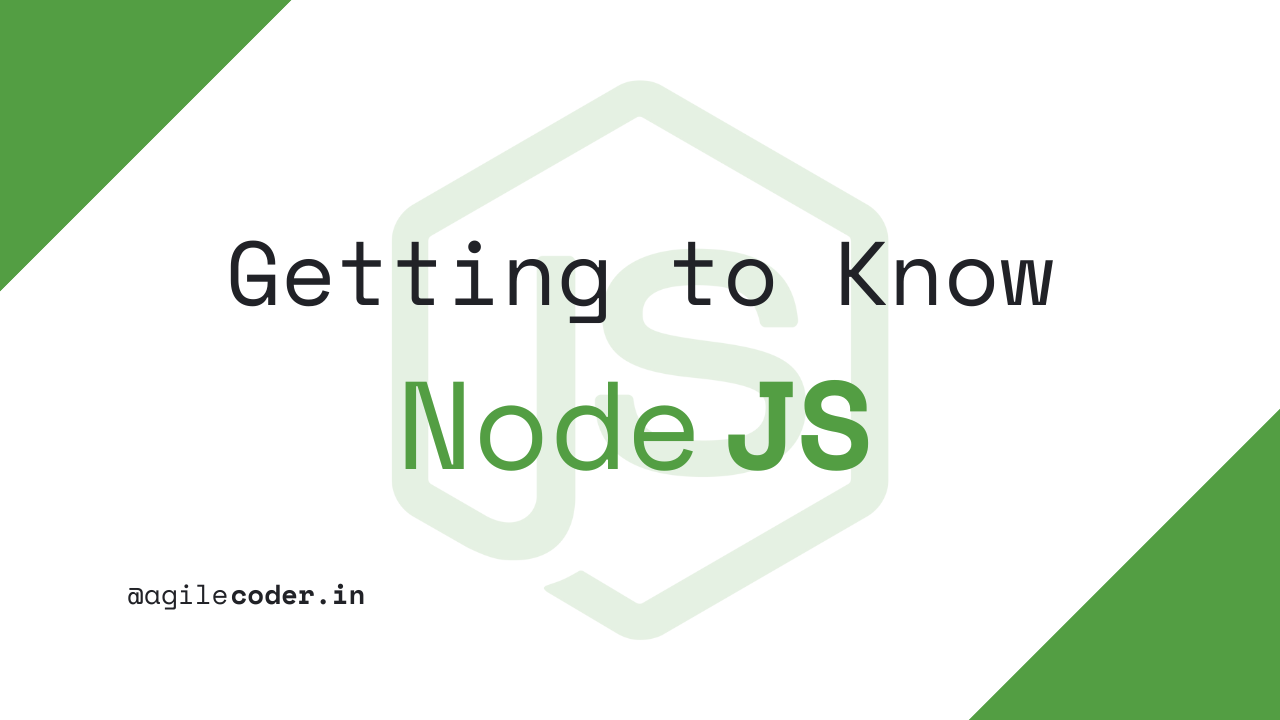
Node.js is a powerful, open-source, server-side platform built on Chrome's V8 JavaScript engine. Known for its speed, efficiency, and scalability, it has become the go-to choice for building fast and scalable network applications. Whether you're just starting or looking to enhance your skills, this hub serves as your comprehensive guide to everything Node.js.
Here, you’ll find tutorials, best practices, tips, and real-world applications that will help you master Node.js from the ground up. Bookmark this page and explore a growing collection of articles that will deepen your understanding of Node.js.
Why Node.js ?
Node.js allows developers to use JavaScript to build server-side applications. Here’s why Node.js is widely used:
- Non-blocking I/O model: Efficient and scalable applications.
- Event-driven architecture: Handles many connections simultaneously.
- JavaScript runtime: Enables developers to use one language across both client and server sides.
- Vast ecosystem: The Node Package Manager (NPM) provides thousands of libraries and tools to simplify development.
What can you build with Node.js ?
With Node.js, you can build:
- Web Servers: Fast, scalable websites.
- Real-time applications: Chat apps, gaming servers.
- RESTful APIs: Lightweight and flexible data interchange systems.
- Microservices: Decoupled services that scale independently.
No matter the project size, Node.js can handle it all with ease.
NodeJS Internals
Do you know what makes you a better software engineer? Understanding the internal architecture and how things work behind the scenes. That's exactly right.
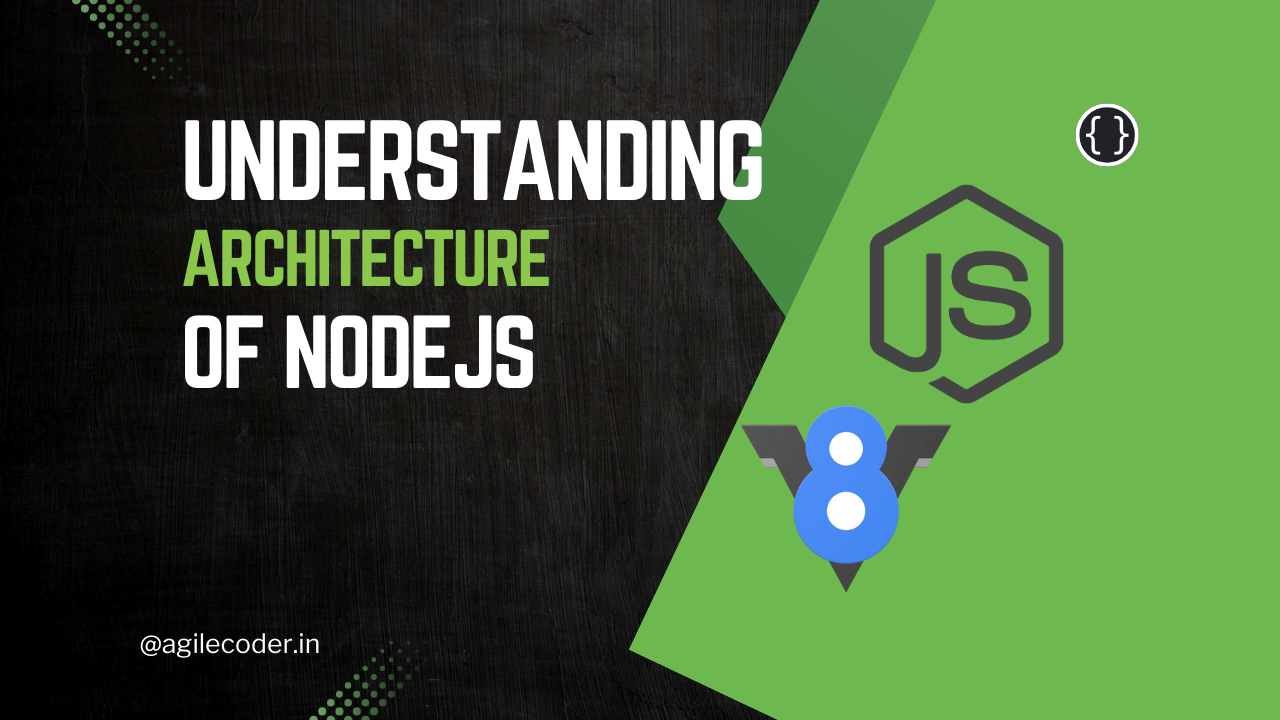
Ready to dive in yet?
Check out the articles below, and don't forget to subscribe to get the latest Node.js tutorials delivered to your inbox!
Web Server Tutorials
Project Setup
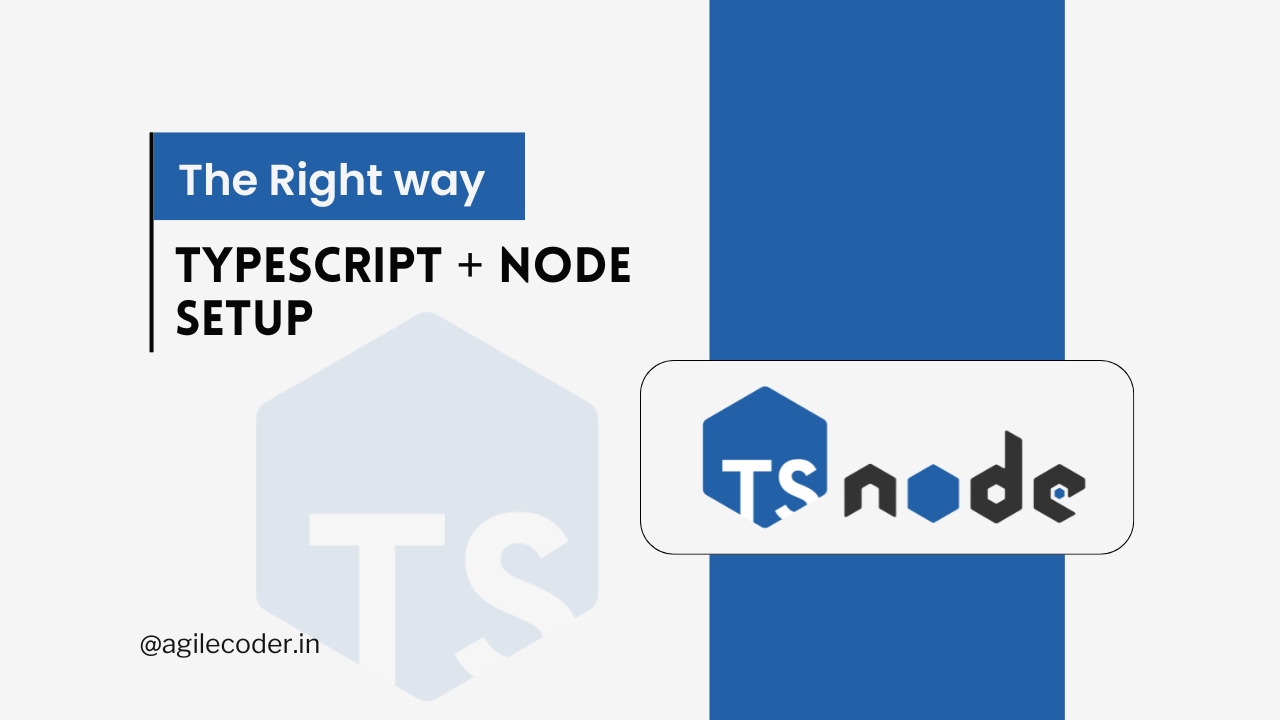
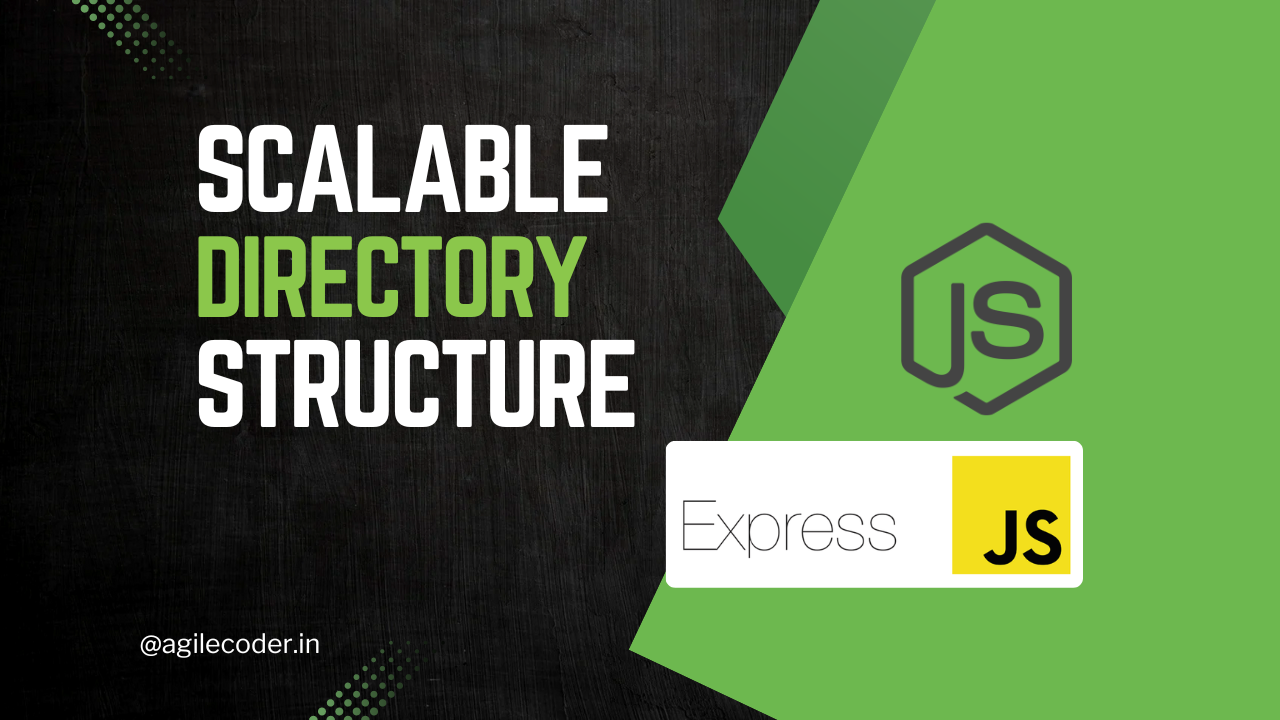
Authentication
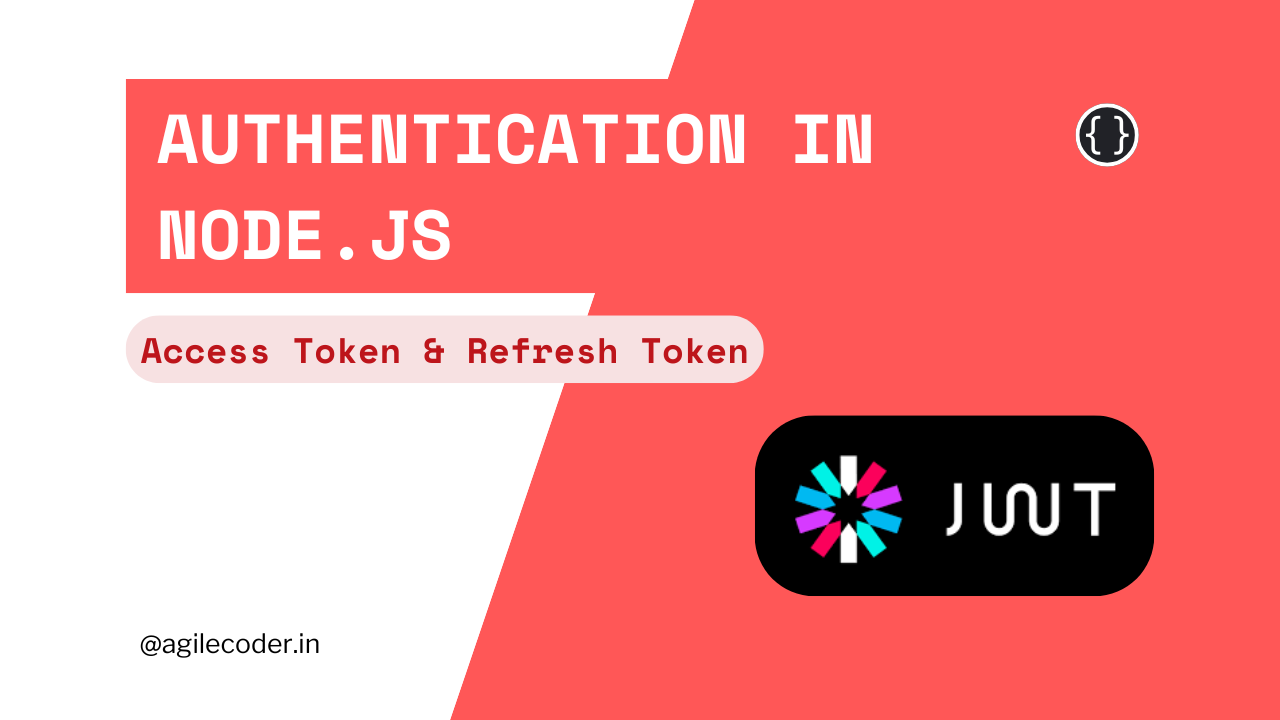
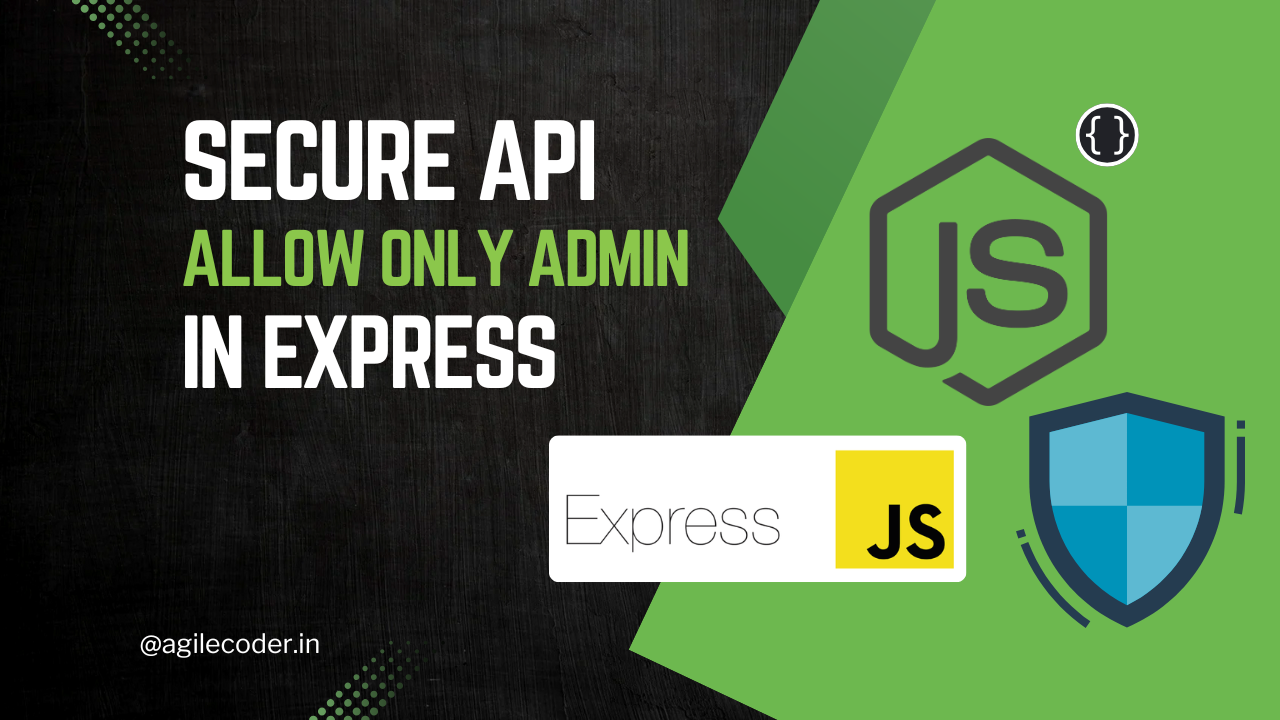
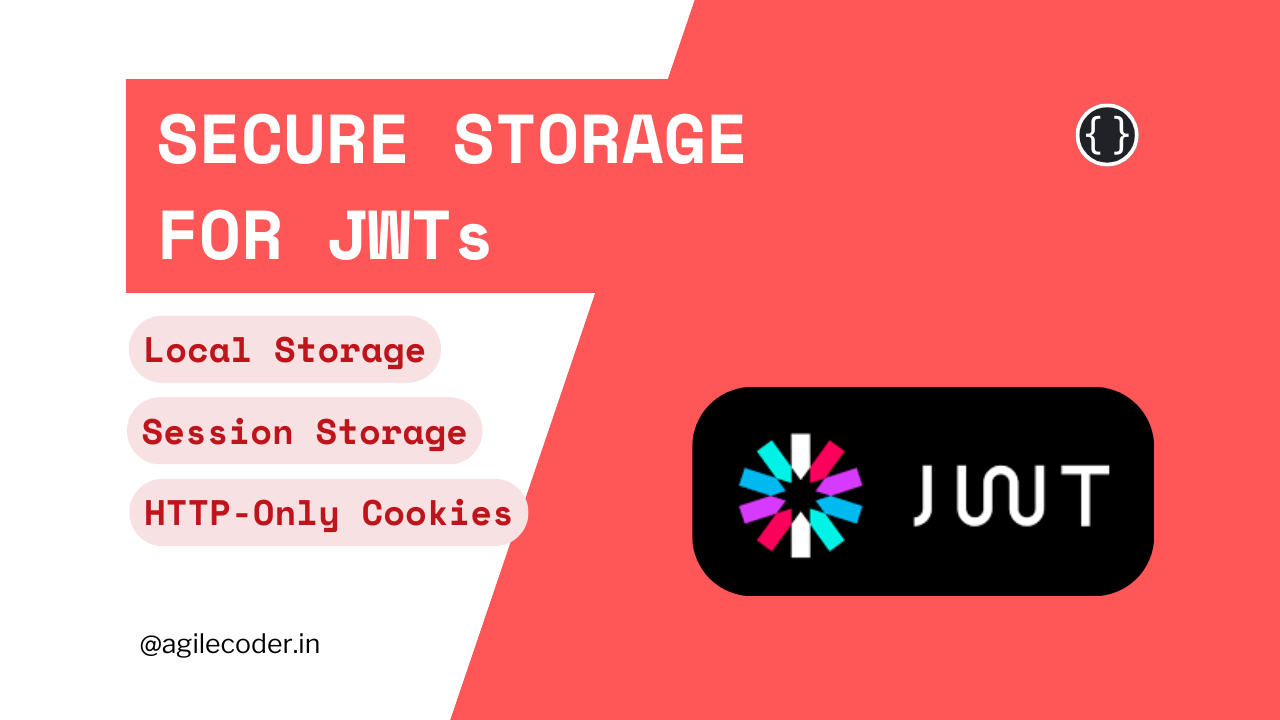
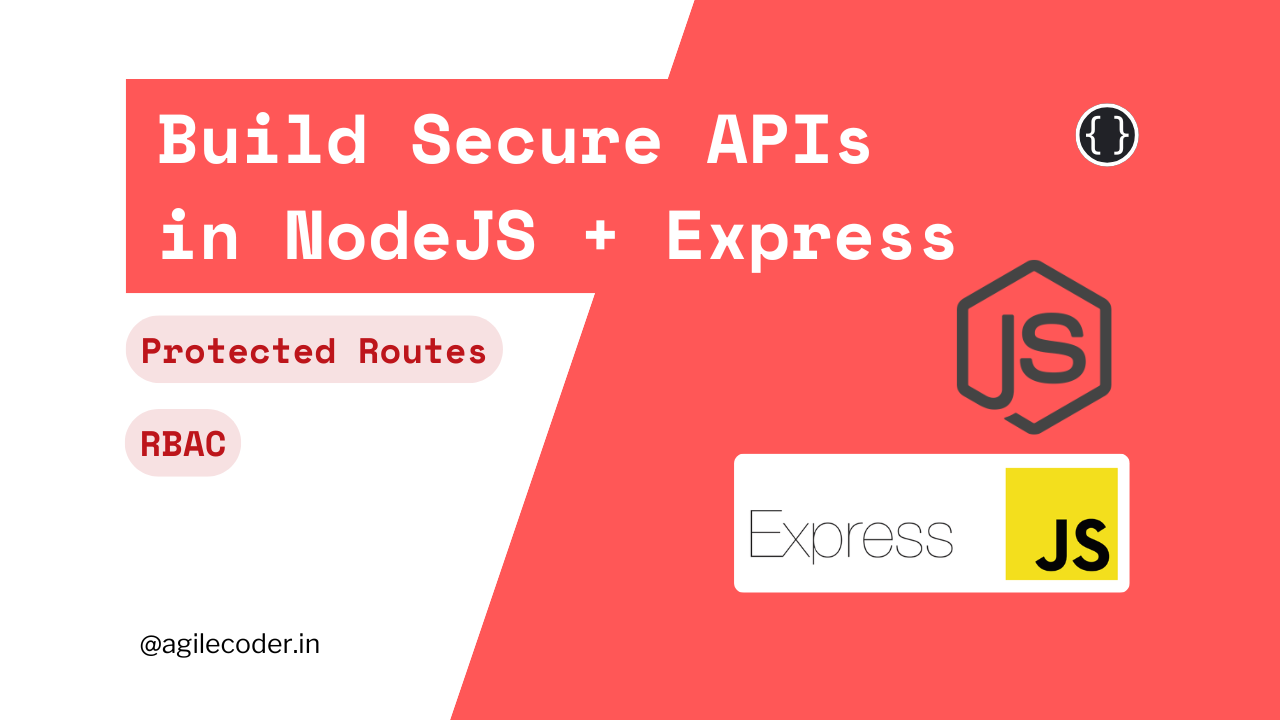
Core Implementations
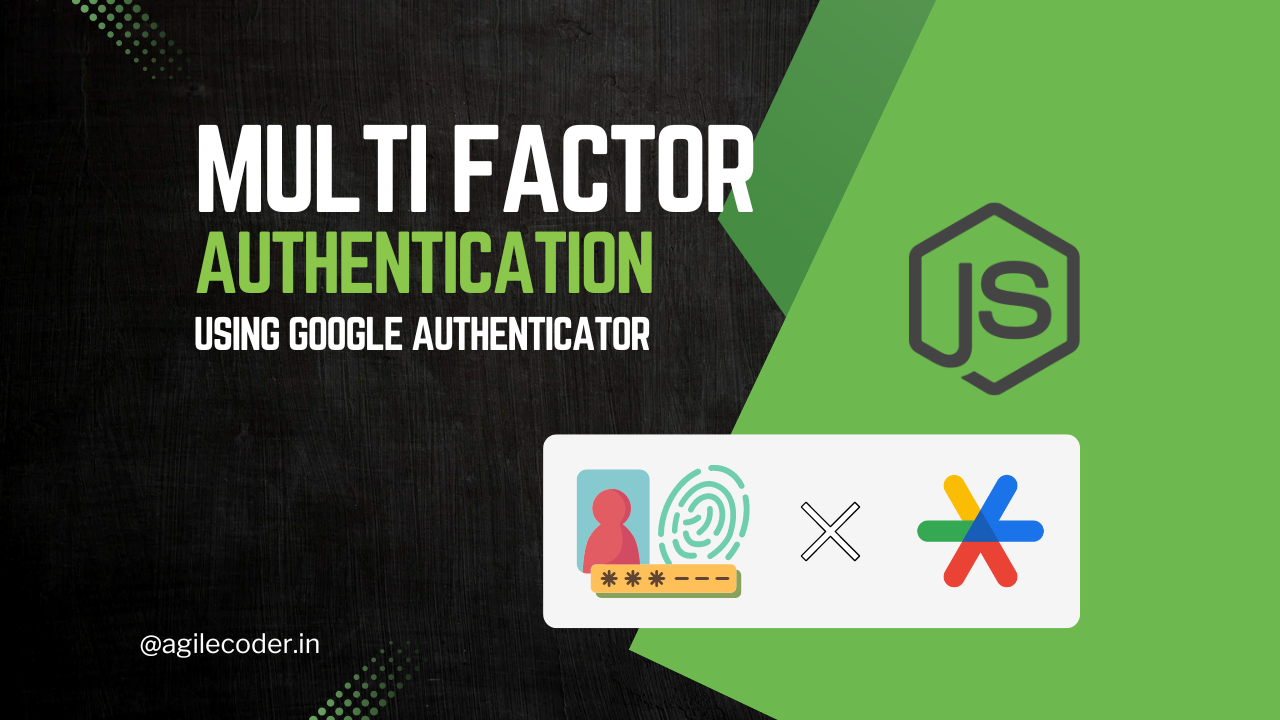
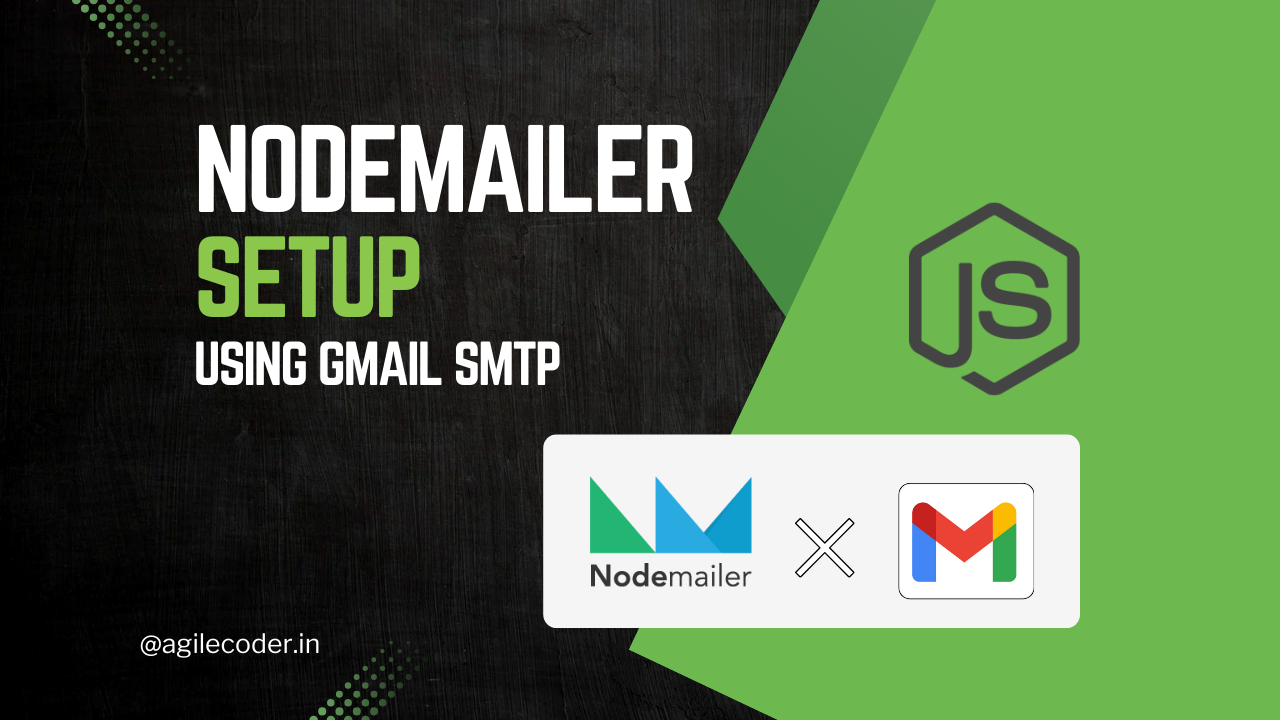
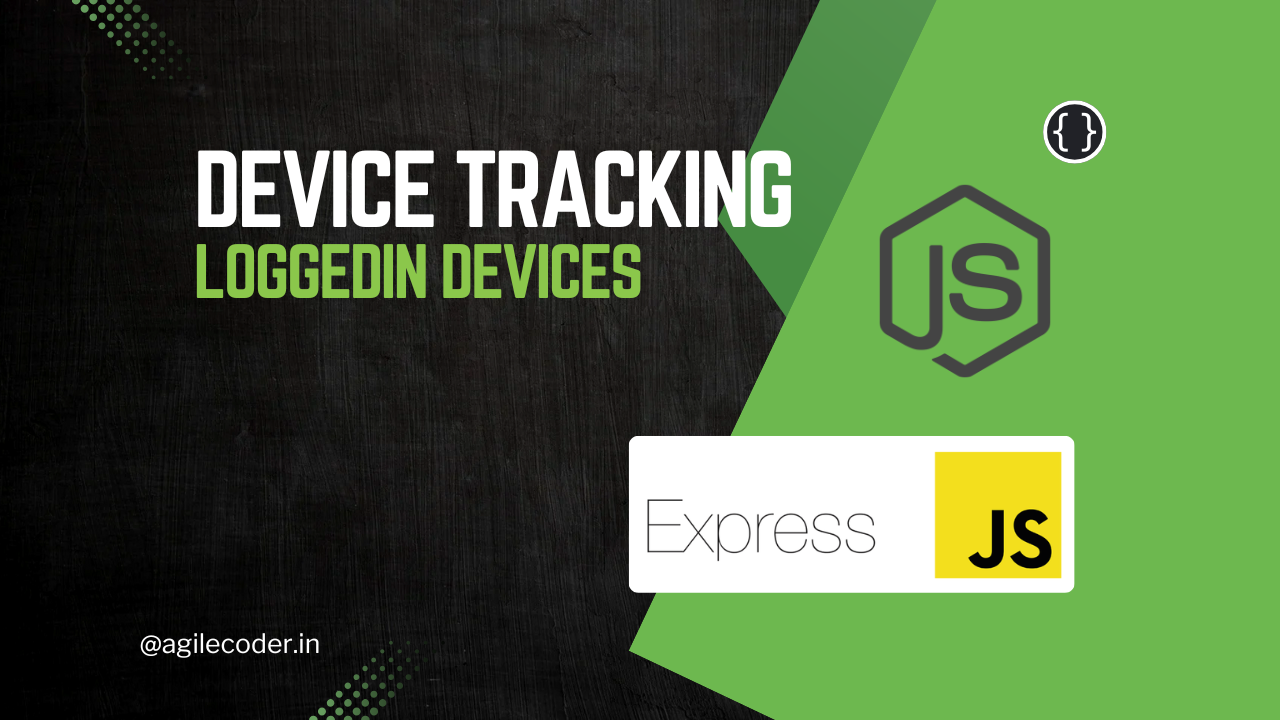
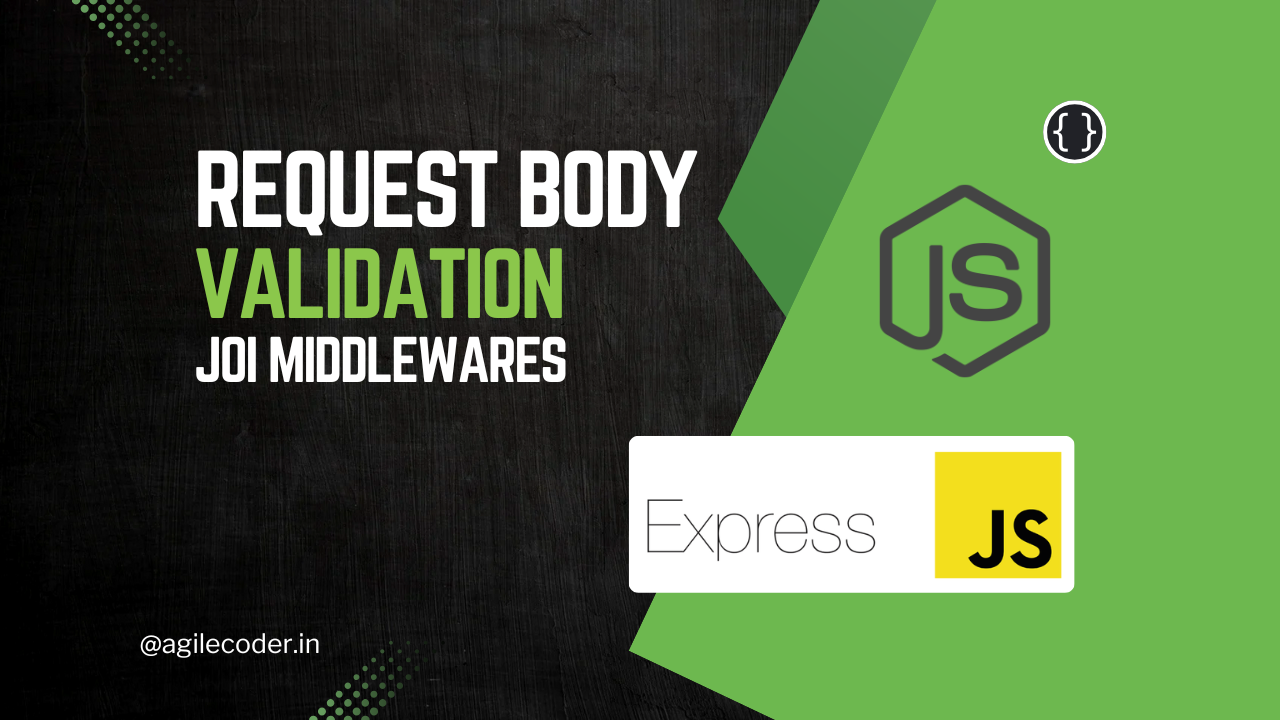
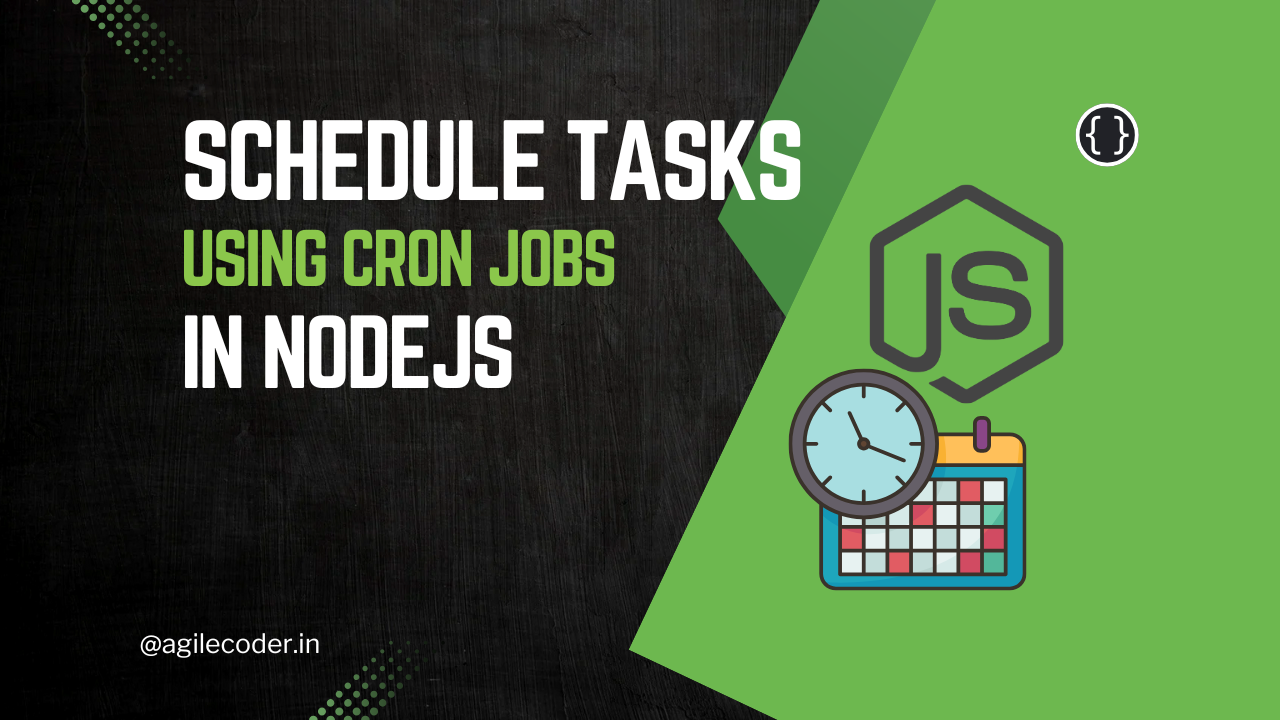
Implementing Cloud Services
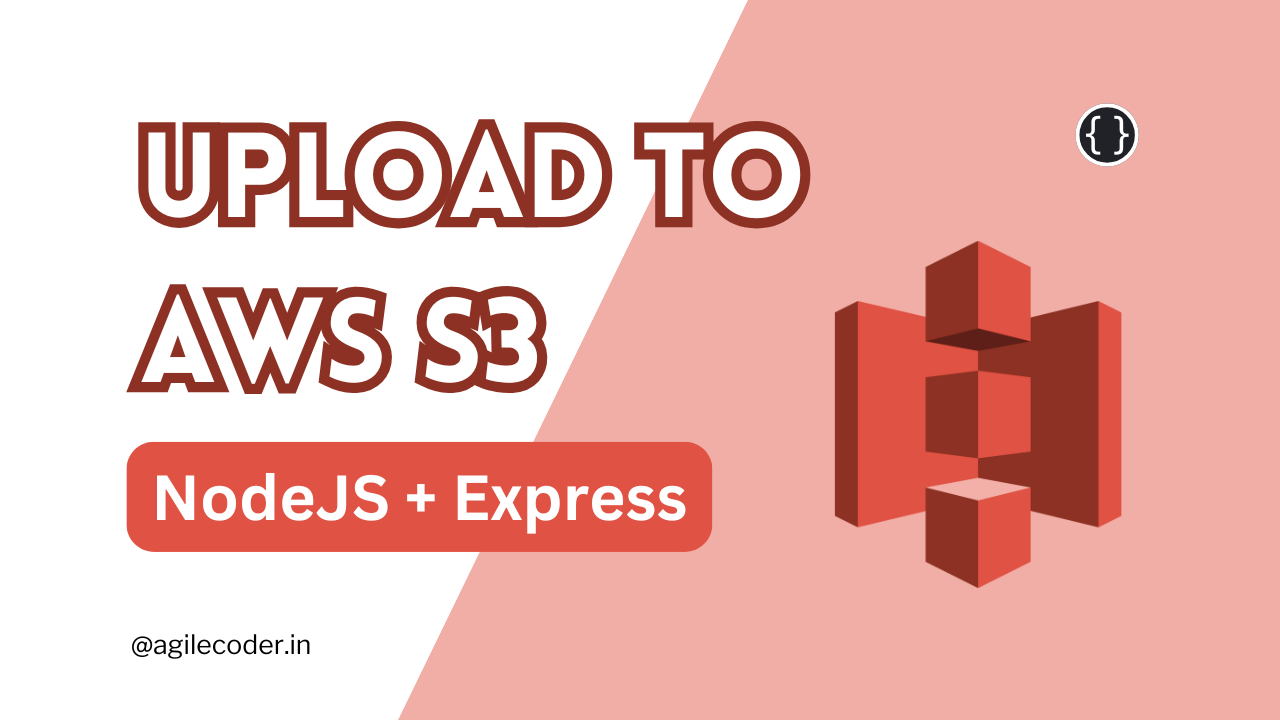
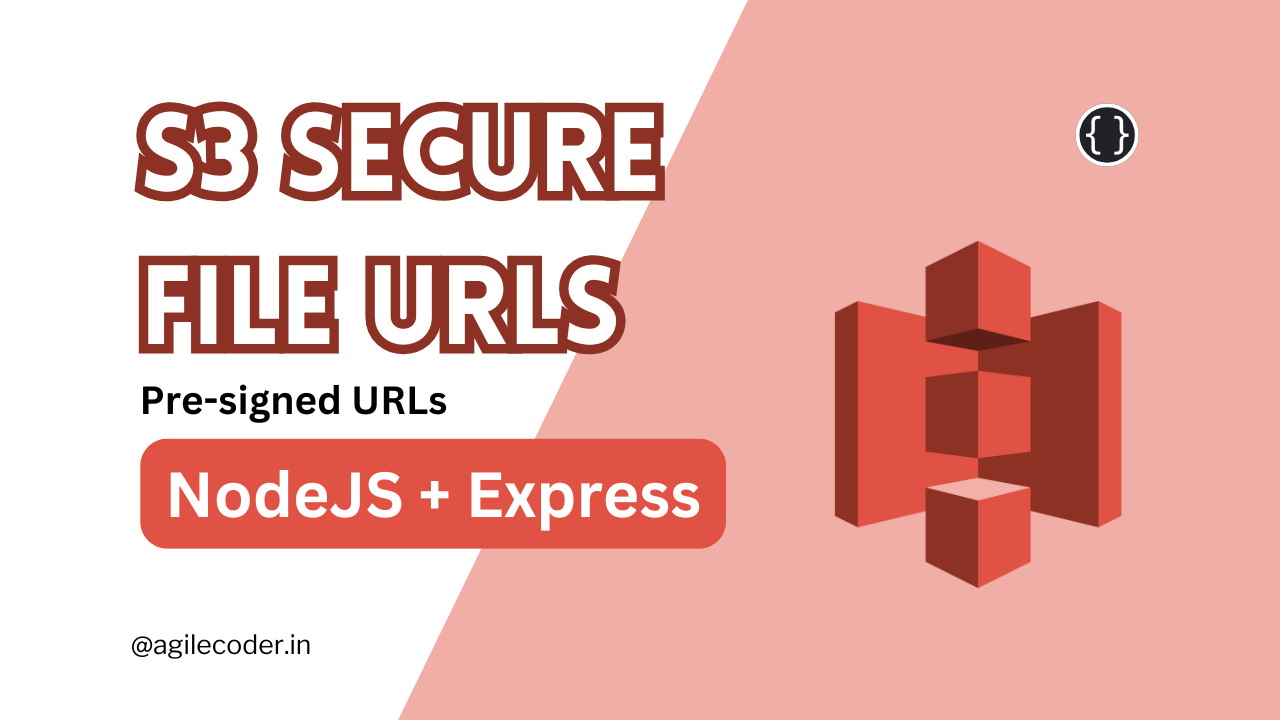
Making Developer's Life Easy
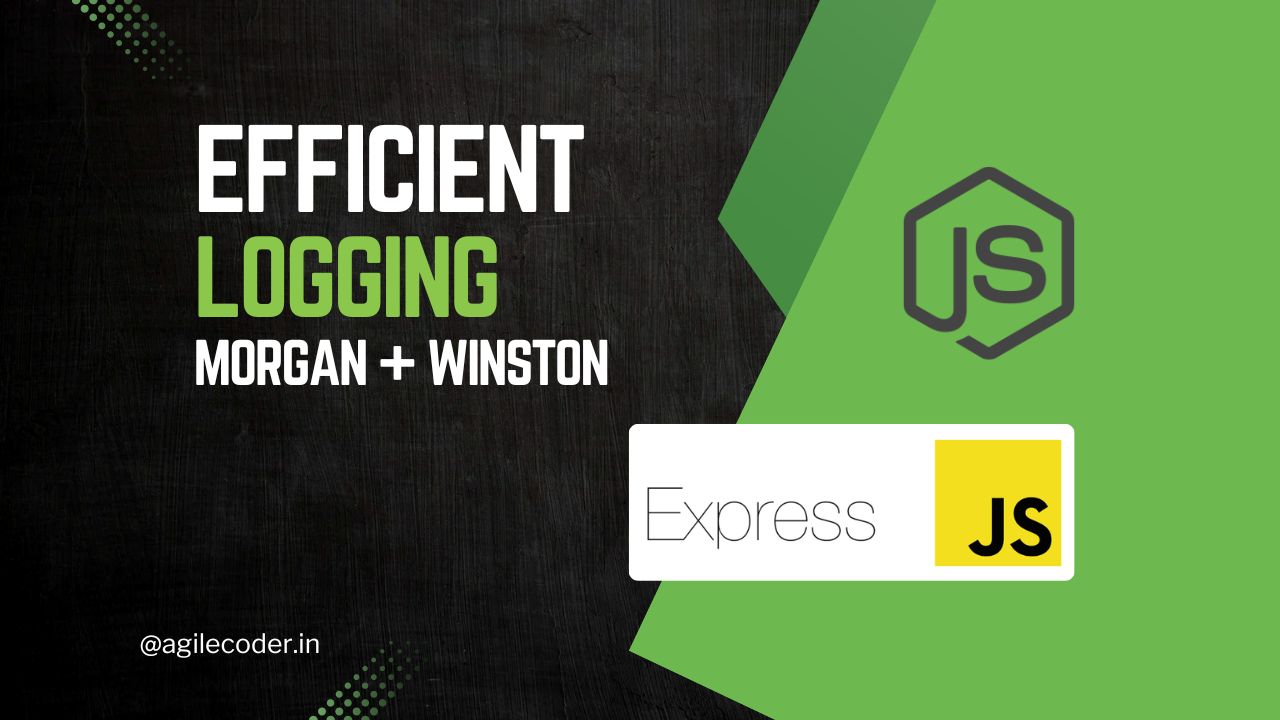
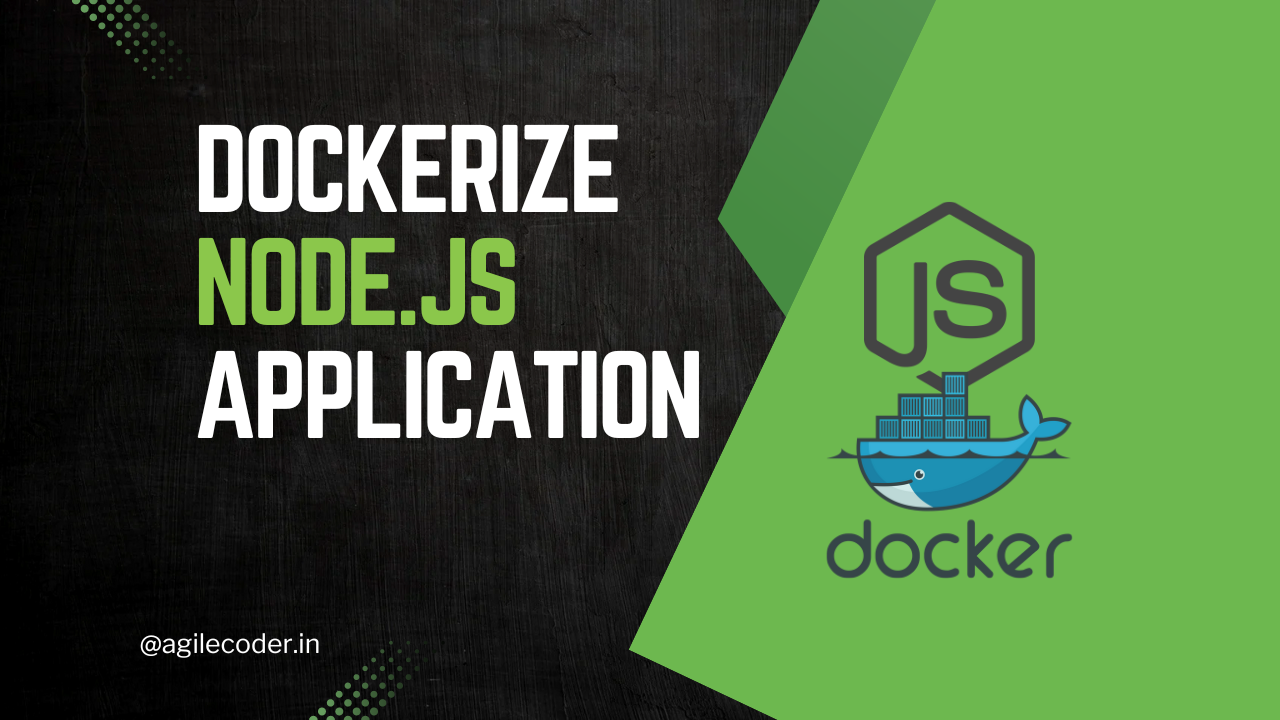
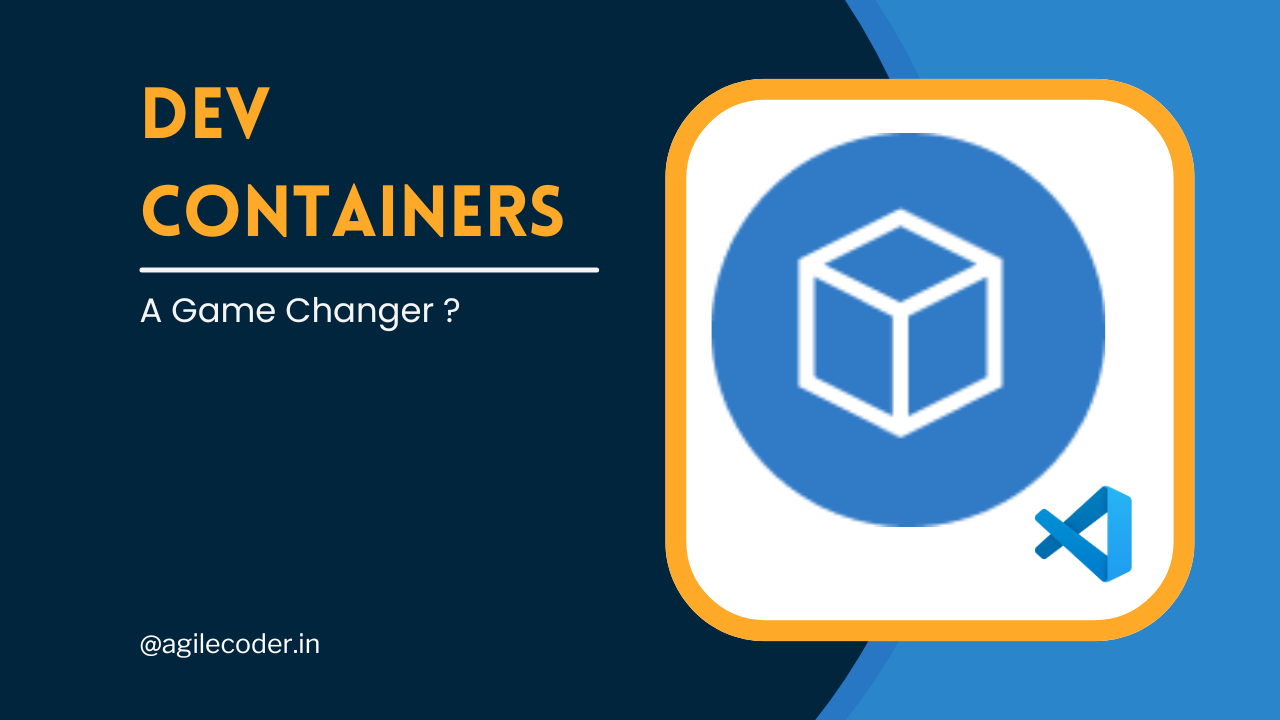