How to Schedule Tasks in Node.js with Cron Jobs
Automate routine tasks in Node.js using node-cron! This guide covers setting up cron jobs to handle data cleanup, external data fetching, and file management. Learn how to create tasks, schedule them with cron expressions, and integrate them into your app to save time and reduce manual work.
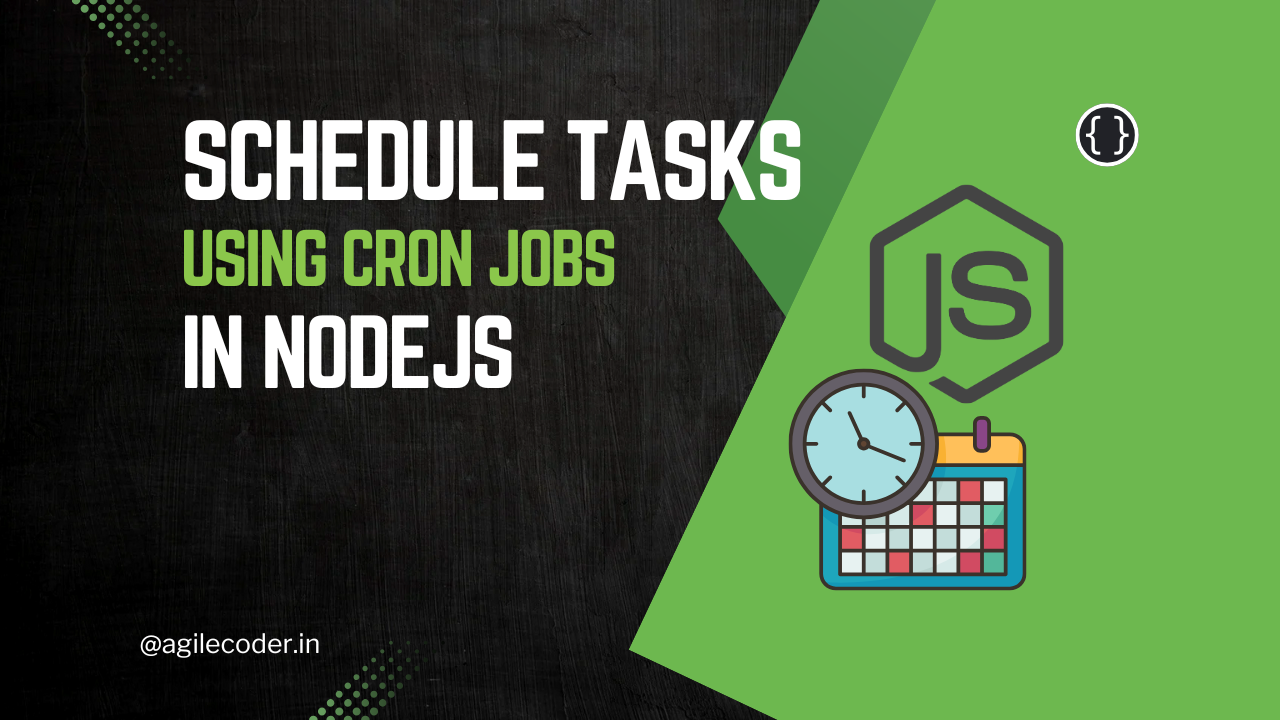
In any application, automating routine tasks like data cleanup, fetching external data, or removing unused files can save time and reduce the risk of human error. In Node.js, we can leverage cron jobs to schedule tasks to run at specified times, making automation seamless. In this tutorial, we’ll learn how to set up and schedule tasks using the popular node-cron
library.
Why Use Cron Jobs?
Cron jobs are commands or scripts that are scheduled to run periodically. They’re ideal for:
- Automating repetitive tasks like data backup or cleanup.
- Scheduling updates, notifications, or other time-based events.
- Executing tasks at off-peak hours to improve performance.
- Cleaning unverified accounts every 30 days.
Prerequisites
To follow along, you’ll need:
- Node.js and npm are installed on your machine.
- Basic knowledge of JavaScript and Node.js.
Step 1: Set Up Your Node.js Project
- Start by creating a new Node.js project:
npm init -y
- Install
node-cron
:
npm install node-cron
node-cron
is a lightweight and easy-to-use library for creating cron jobs in Node.js. It allows you to define cron expressions, which determine the schedule for each job.
Step 2: Define the Scheduled Tasks
In a real-world application, you may have tasks such as data fetching, cleaning up unused files, or managing expired records. Let’s look at an example where we define a few tasks:
- Fetching Tor Exit Nodes (for network-related tasks)
- Deleting Trashed Items (for database cleanup)
- Deleting Unused Attachments (for file management)
Let’s create a folder named jobs
to house our task files.
Create Task Files
In the jobs
folder, add the following files for our example tasks:
fetchTorNodes.js
– simulates fetching Tor exit nodes.
export const fetchTorExitNodes = () => {
console.log("Fetching Tor exit nodes...");
// Add Logic
};
deleteTrashedItems.js
– simulates deleting trashed items.
export const deleteTrashedItems = () => {
console.log("Deleting trashed items...");
// Add Logic
};
deleteUnusedAttachments.js
– simulates deleting unused attachments.
export const deleteUnusedAttachments = () => {
console.log("Deleting unused attachments...");
// Add Logic
};
Our cron job scheduler will call these tasks at specific times. The tasks can be anything you want to happen repetitively over a certain interval of time.
Step 3: Set Up the Cron Scheduler
Now, we’ll create a cron.js
file to configure the cron jobs.
In this example, we’ll set up three different schedules:
- Fetch Tor exit nodes every 12 hours.
- Delete trashed items daily at midnight.
- Delete unused attachments daily at midnight.
import cron from "node-cron";
import { fetchTorExitNodes } from "./jobs/fetchTorNodes";
import { deleteTrashedItems } from "./jobs/deleteTrashedItems";
import { deleteUnusedAttachments } from "./jobs/deleteUnusedAttachments";
const immediateJobs = () => {
fetchTorExitNodes(); // Run immediately upon server start
};
export const cronJobs = () => {
cron.schedule("0 */12 * * *", fetchTorExitNodes); // Every 12 hours
cron.schedule("0 0 * * *", deleteTrashedItems); // Every day at midnight
cron.schedule("0 0 * * *", deleteUnusedAttachments); // Every day at midnight
immediateJobs(); // Run immediate jobs on start
};
Explanation
Each job is scheduled with a cron expression. Let’s break down "0 */12 * * *"
:
0
: Execute in the 0th minute.*/12
: Run every 12 hours.* * *
: Run every day, every month, regardless of the weekday.
Similarly, "0 0 * * *"
runs daily at midnight.
Step 4: Integrate the Cron Scheduler into Your Application
Now that we have the scheduler configured, let’s integrate it into our main server file.
- Create a new file
server.js
, or modify your existing server file:
import { connectDatabase } from "./configs/db";
import app from "./server";
import dotenv from "dotenv";
import { cronJobs } from "./cron";
import logger from "./configs/logger";
dotenv.config();
const PORT = Number(process.env.PORT) || 3000;
connectDatabase(() => {
app.listen(PORT, () => {
logger.info(`Server is running at http://localhost:${PORT} 🚀`);
cronJobs(); // Initialize cron jobs when the server starts
});
});
In this setup, we import and call cronJobs()
right after starting the server. This way, our cron jobs are active as soon as the server is up and running.
Step 5: Test the Cron Jobs
To test your cron jobs:
- Start the server:
node server.js
- You should see a message like
Fetching Tor exit nodes...
, because it is an immediate job.
Additional Tips for Using Cron Jobs in Node.js
- Logging: Use a logging library to log cron job activity, which can be useful for monitoring task execution.
- Error Handling: Wrap task functions in try-catch blocks or use async error handling to avoid crashes if a job fails.
- Dynamic Scheduling: Use environment variables or a configuration file to set cron schedules dynamically.
Cron jobs are a powerful tool for automating tasks in Node.js. With node-cron
, we can quickly set up and manage these jobs, making our applications more efficient and reliable. Whether you’re handling database cleanup, fetching external data, or removing unused files, using cron jobs allows you to easily automate tasks.
Explore more complex cron expressions, handle failures gracefully, and consider integrating more sophisticated job schedulers like Agenda
or Bree
if you need advanced features like job queuing or database-backed scheduling.
That's it for this article! See ya 👋