Collect and Handle Request Details in a Node.js + Express Server
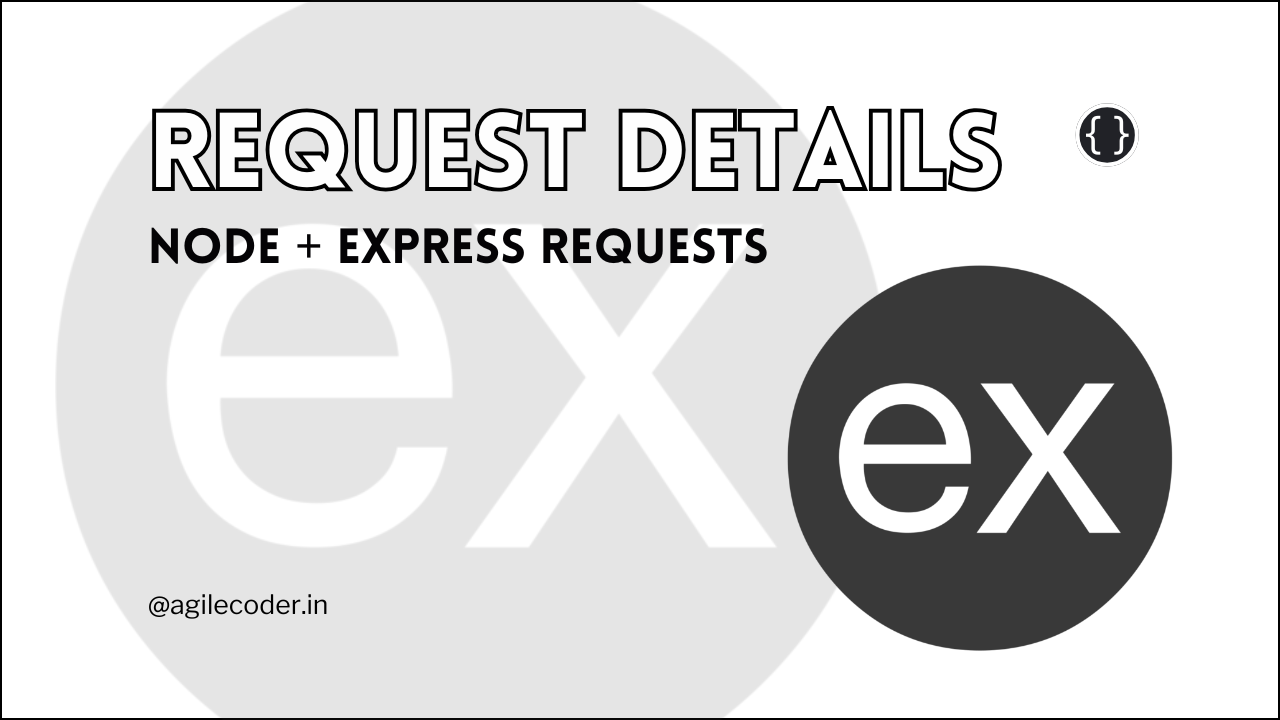
In web development, understanding client information can be crucial for enhancing user experience, security, and debugging. When working with a Node.js and Express server, collecting and handling request details like the client’s IP address, browser type, operating system, and device type can provide valuable insights.
This blog post demonstrates how to implement a middleware function in Express to collect these request details using the express-useragent
and request-ip
libraries. We’ll walk through a code snippet that gathers client-specific information and attaches it to the request object for further processing.
All links can be found in the resources section at the bottom of the article
Prerequisites
Before diving into the code, make sure you have the following:
- Node.js and npm installed on your machine.
- An existing Express application.
- Install the required libraries by running:
npm install express-useragent request-ip
Implementation
We can create a middleware and attach it to requests, for which we want to collect data. Here is the snippet that can work for you:
import { Request, Response, NextFunction } from "express";
import useragent from "express-useragent";
import requestIp from "request-ip";
import { ExtendedRequest } from "../domains/user/types";
const collectRequestDetails = (req: Request, res: Response, next: NextFunction) => {
const clientIp = requestIp.getClientIp(req);
const ua = useragent.parse((req as any).headers["user-agent"]);
(req as ExtendedRequest).requestDetails = {
ip: clientIp || "",
browser: ua.browser,
browser_version: ua.version,
os: ua.os,
platform: ua.platform,
is_mobile: ua.isMobile,
is_desktop: ua.isDesktop,
is_tablet: ua.isTablet,
};
next();
};
export default collectRequestDetails;
Explanation
1. Dependencies
request-ip
: Extracts the client's IP address from the request.express-useragent
: Parses theUser-Agent
header to gather browser and device information.
2. Middleware Function
- The
collectRequestDetails
middleware extracts details about the client and attaches them to therequest
object.
3. ExtendedRequest
To add a custom property (requestDetails
) to the Request
object, we use TypeScript's type extension. Here’s an example of how the ExtendedRequest
type could look:
export interface ExtendedRequest extends Request {
requestDetails?: {
ip: string;
browser: string;
browser_version: string;
os: string;
platform: string;
is_mobile: boolean;
is_desktop: boolean;
is_tablet: boolean;
};
}
4. Data Collected
- IP Address: Using
request-ip
to get the client’s IP address. - Browser Information: Includes browser name and version.
- Operating System: Identifies the client’s OS.
- Device Type: Flags whether the client is on mobile, desktop, or tablet.
5. Passing Control
The next()
function ensures that the request proceeds to the next middleware or route handler in the stack.
Using the Middleware
To use this middleware in your Express application, simply include it in your routes or globally:
Global Middleware
import express from "express";
import collectRequestDetails from "./middlewares/collectRequestDetails";
const app = express();
app.use(collectRequestDetails);
app.get("/", (req, res) => {
res.json((req as ExtendedRequest).requestDetails);
});
app.listen(3000, () => {
console.log("Server is running on port 3000");
});
Route-Specific Middleware
app.get("/info", collectRequestDetails, (req, res) => {
res.json((req as ExtendedRequest).requestDetails);
});
Below is the response you can get
{
"ip": "203.0.113.195",
"browser": "Chrome",
"browser_version": "117.0.0.1",
"os": "Windows",
"platform": "Microsoft Windows",
"is_mobile": false,
"is_desktop": true,
"is_tablet": false
}
Benefits
- Enhanced Debugging: Understand client environments better when troubleshooting issues.
- User Segmentation: Tailor experiences based on the client’s device or browser.
- Security: Log IPs for suspicious activity detection.
By implementing the collectRequestDetails
middleware, you can gain valuable insights into client requests in a Node.js + Express server. This information can be used for debugging, enhancing user experience, or improving security.
Feel free to adapt this code to fit your project’s specific requirements!
That's it for today. See ya 👋
Resources
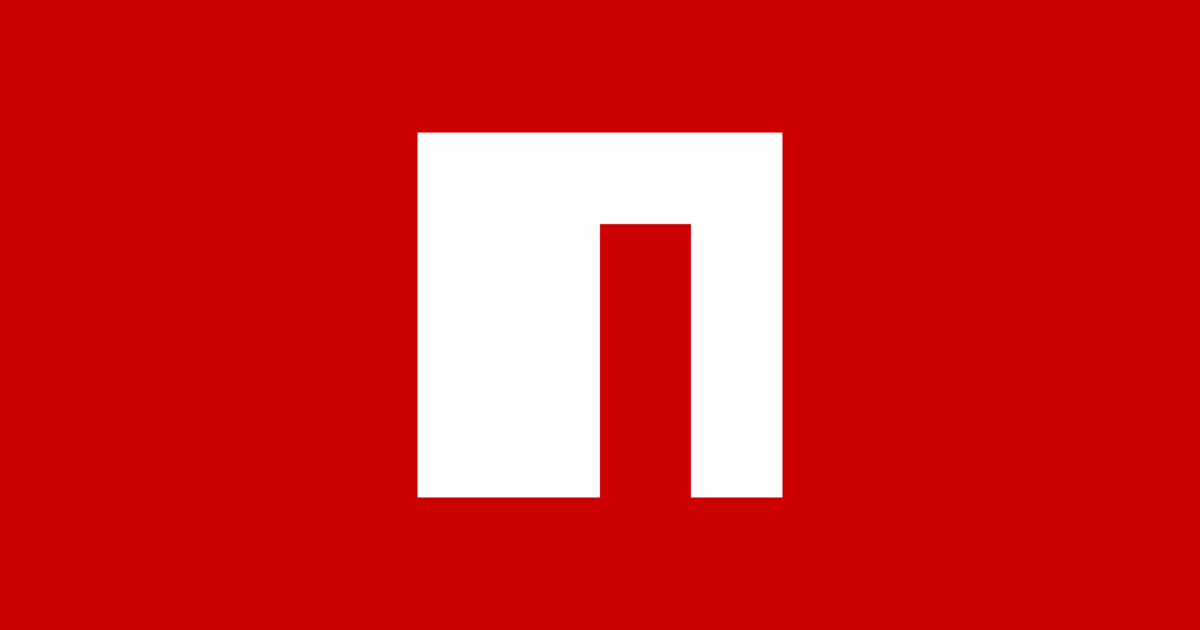
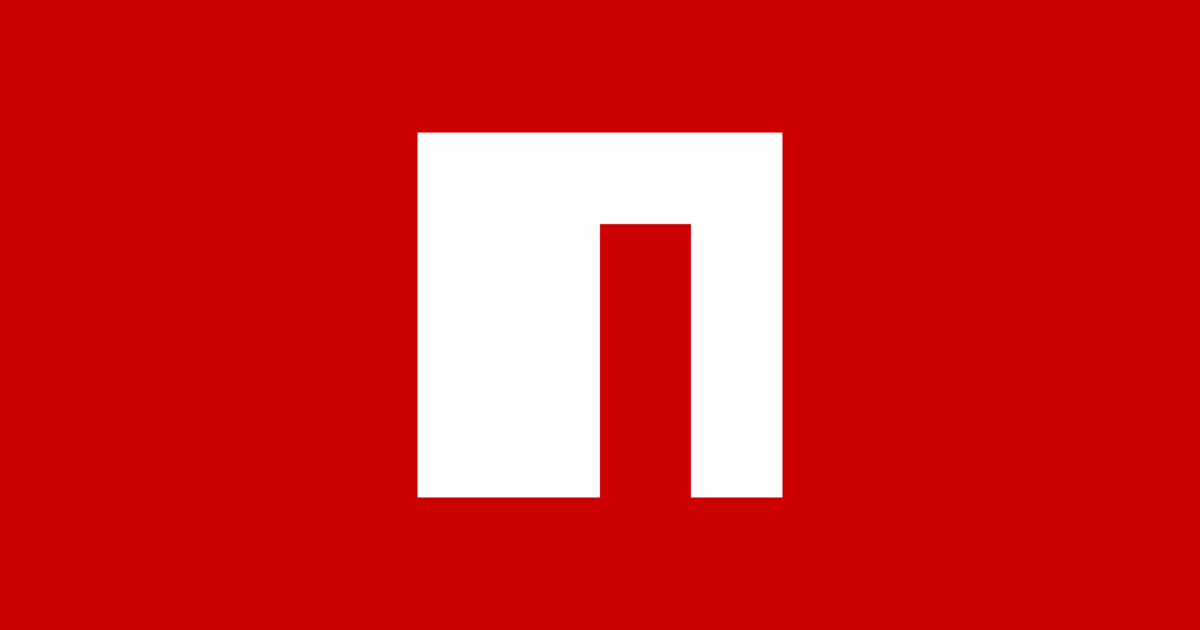